True
14 Dimensionality Reduction
14.1 PCA
Examples
We next consider an image approximation (or compression) example where matrix basis are used. Consider the following image:
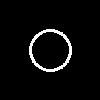
Figure 14.1 shows an image of a circle, which is \(100 \times 100\) in size. While the image is in 2D, the pixel space is \(\mathcal{R}^{100\times 100}\), which needs \(100\times 100=10000\) basis matrices!
However, looking at the image, we can see that most of the image is empty (black pixels) and the circle can be represented with much smaller number of basis functions. Thus, by inspection, we can infer that the image can be represented in a lower dimensional space. We can apply singular value decomposition to determine lower dimensional (or reduced order) representation of the image. This is also known as image compression.
The following Python code demonstrates it.
import numpy as np
import matplotlib.pyplot as plt
from scipy.linalg import svd
from PIL import Image
# Load the image into a numpy array.
= Image.open('images/circle.png')
img
# Perform SVD
= svd(img)
U, Sigma, Vt
= plt.stem(range(len(Sigma)),Sigma)
markerline, stemline, baseline = 3)
plt.setp(markerline, markersize "Singular values of the image matrix.")
plt.title("Index k.")
plt.xlabel("Singular Value") plt.ylabel(
Text(0, 0.5, 'Singular Value')
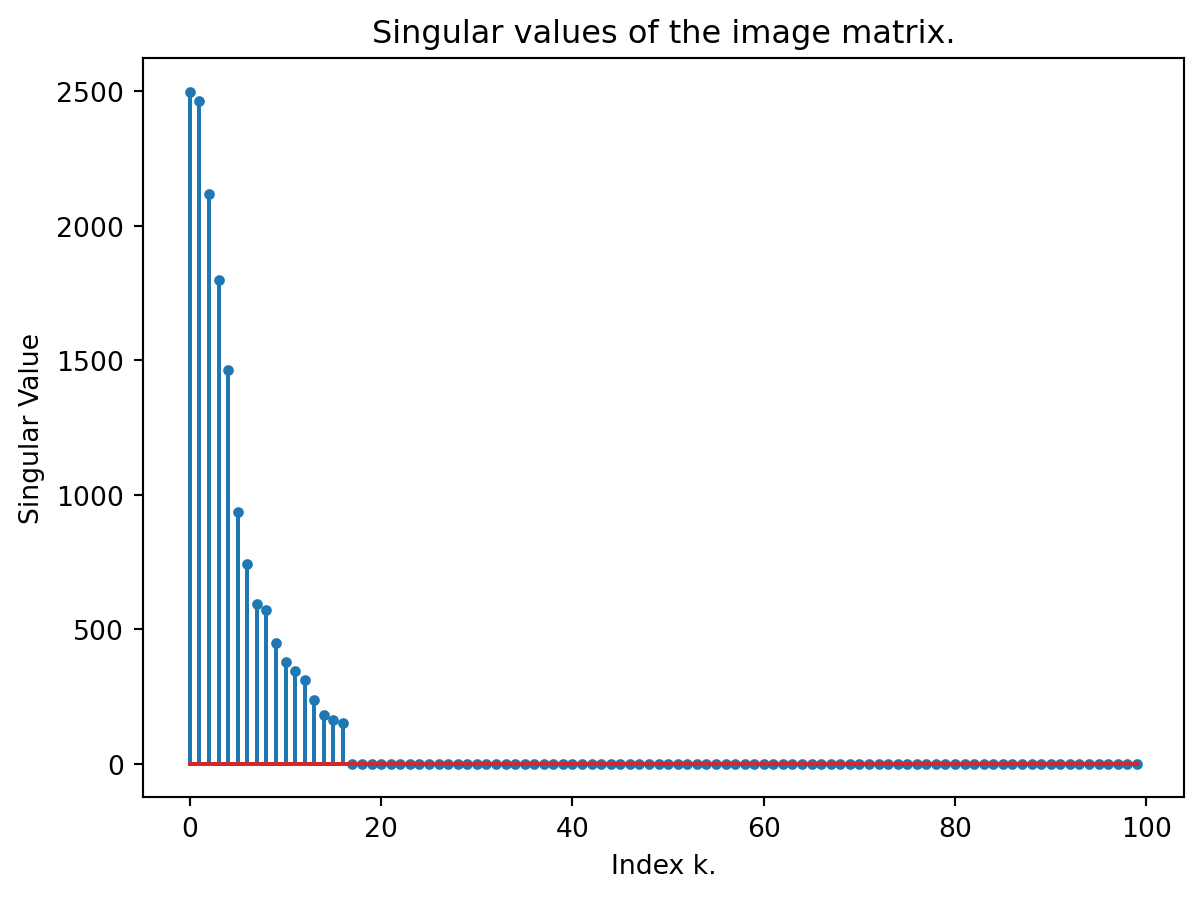
Figure 14.2 shows the singular values of the image matrix. We observe that most of the singular values are zero, indicating the image can be represented in a much lower dimensional space. The next Python code shows how it can be done.
def compress_image(U,Vt,Sigma,k):
= U[:, :k]
U_k = np.diag(Sigma[:k])
Sigma_k = Vt[:k, :]
Vt_k = np.dot(U_k, np.dot(Sigma_k, Vt_k))
A_k print(U_k.shape)
return A_k
= compress_image(U,Vt,Sigma,20)
img1 = compress_image(U,Vt,Sigma,10)
img2 = compress_image(U,Vt,Sigma,5)
img3
# Plot the original and the compressed image
plt.figure()2, 2, 1)
plt.subplot(='gray')
plt.imshow(img, cmap'Original Image')
plt.title(
2, 2, 2)
plt.subplot(='gray')
plt.imshow(img1, cmap'Compressed Image with k=20')
plt.title(
2, 2, 3)
plt.subplot(='gray')
plt.imshow(img2, cmap'Compressed Image with k=10')
plt.title(
2, 2, 4)
plt.subplot(='gray')
plt.imshow(img3, cmap'Compressed Image with k=5')
plt.title(
plt.tight_layout() plt.show()
(100, 20)
(100, 10)
(100, 5)